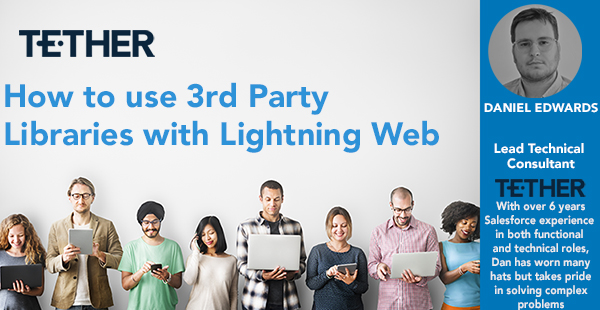
We’ve all been there.
Pining for a chance to use some of the cool JavaScript libraries like jQuery, MomentJS or Chart.js? Well now you can!
The LWC framework makes it easy to use external JavaScript libraries to expand functionality beyond what Salesforce can provide, take Two.js for example – Two.js is a 2d graphics library that allows the drawing and connecting of shapes, ideal for ERD projects or visualisation.
Enough chit chat, let’s see how it works!
Step 1: Uploading Your JavaScript Library as a Static Resource
The first step is to upload our JavaScript library as a Static Resource within Salesforce, lets do it:
- Click Setup
- Search ‘Static Resource’
- Click ‘New’, upload your JavaScript file and name it
Step 2: Generating the LWC Component
Once the external JavaScript library has been uploaded as a Static Resource, we can move onto generating the LWC:
import { LightningElement, api, track } from 'lwc';
import { loadScript } from 'lightning/platformResourceLoader';
import TwoJs from '@salesforce/resourceUrl/TwoJs';
export default class TwoJsWrapper extends LightningElement {
@api width;
@api height;
twoJsInitialized = false;
renderedCallback() {
if (this.twoJsInitialized) {
return;
}
this.twoJsInitialized = true;
loadScript(this, TwoJs)
.then(() => {
this.initializeTwoJs();
})
.catch((error) => {
console.error('Error loading Two.js', error);
});
}
initializeTwoJs() {
const container = this.template.querySelector('.two-js-container');
const two = new Two();
two.width = this.width || 400;
two.height = this.height || 300;
two.appendTo(container);
// Use Two.js to create your graphics and animations
// Example: Create a red circle
const circle = two.makeCircle(two.width / 2, two.height / 2, 50);
circle.fill = 'red';
two.update();
}
}
Step 3: Using the loadScript Function
In order to load external JavaScript libraries, we need to use the loadScript function of the platform resource loader, this is what allows us to safely import JavaScript in a controlled way.
The loadScript function must be called and handled with a callback, the callback allows the use of methods:
loadScript(this, TwoJs)
.then(() => {
this.initializeTwoJs();
// Do some logic!
})
.catch((error) => {
console.error('Error loading Two.js', error);
});
In the full example above, we load in Two.js using the loadScript function, we then specify where the drawing area will be (A div with a class name of ‘.two-js-container’) and then we create a red circle to display within the page.
Conclusion
And that is really how simple it is! LWC is much more tolerant of external JavaScript libraries and as long as it is reputable, you can use anything you like.
Thanks for reading,
Daniel Edwards
The Tether Team
#salesforce #salesforcecrm #tethertips #tether #code #3rdparty